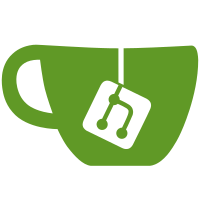
removed unnecessary components, combined where necessary added each component to a folder, added individual css style modules optimized some component rendering flows removed functional components in favor of pure components linted and formatted all of the files
53 lines
1.3 KiB
JavaScript
53 lines
1.3 KiB
JavaScript
import { FormattedMessage, injectIntl, defineMessages } from 'react-intl';
|
|
import classNames from 'classnames';
|
|
import Icon from '../icon';
|
|
|
|
import './index.scss';
|
|
|
|
const messages = defineMessages({
|
|
load_more: { id: 'status.load_more', defaultMessage: 'Load more' },
|
|
});
|
|
|
|
export default @injectIntl
|
|
class LoadMore extends PureComponent {
|
|
|
|
static propTypes = {
|
|
onClick: PropTypes.func,
|
|
disabled: PropTypes.bool,
|
|
visible: PropTypes.bool,
|
|
maxId: PropTypes.string,
|
|
gap: PropTypes.bool,
|
|
intl: PropTypes.object.isRequired,
|
|
}
|
|
|
|
static defaultProps = {
|
|
visible: true,
|
|
}
|
|
|
|
handleClick = () => {
|
|
const { gap, maxId } = this.props;
|
|
this.props.onClick(gap ? maxId : undefined);
|
|
}
|
|
|
|
render() {
|
|
const { disabled, visible, gap, intl } = this.props;
|
|
|
|
const btnClasses = classNames('load-more', {
|
|
'load-more--gap': gap,
|
|
});
|
|
|
|
return (
|
|
<button
|
|
className={btnClasses}
|
|
disabled={disabled || !visible}
|
|
style={{ visibility: visible ? 'visible' : 'hidden' }}
|
|
onClick={this.handleClick}
|
|
aria-label={intl.formatMessage(messages.load_more)}
|
|
>
|
|
{!gap && <FormattedMessage id='status.load_more' defaultMessage='Load more' />}
|
|
{gap && <Icon id='ellipsis-h' />}
|
|
</button>
|
|
);
|
|
}
|
|
|
|
} |